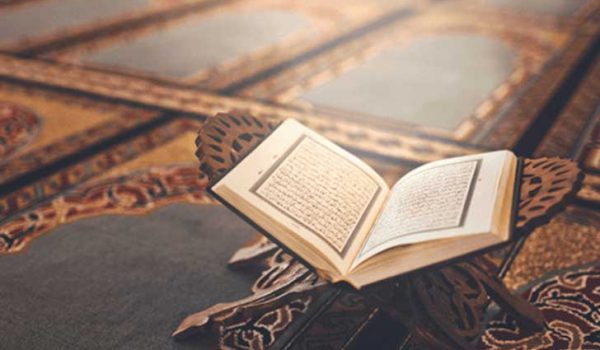
سیدمهدی سیف/ حروف ساکن
در این برنامه استاد سید مهدی سیف درباره موضوع حروف ساکن توضیح می دهند همچنین به مرور دروس گذشته می پردازند.
در این برنامه استاد سید مهدی سیف درباره موضوع حروف ساکن توضیح می دهند همچنین به مرور دروس گذشته می پردازند.
در این برنامه استاد سید مهدی سیف درباره موضوع الفی که در هفت کلمه قران ناخوانا است توضیح می دهند همچنین به مرور دروس گذشته می پردازند.تعریف حروف ناخوانادر کلمه…
در این برنامه استاد سید مهدی سیف درباره موضوع تنوین و الف وصل توضیح می دهند همچنین به مرور دروس گذشته می پردازند.تنوینها فقط با آخرین حرف کلمه همراه می…
در این برنامه استاد سید مهدی سیف درباره موضوع حروف ناخوانا و موارد استثنای لام توضیح می دهند همچنین به مرور دروس گذشته می پردازند.دو نکته: برای شنیدن صدای یک…
در این برنامه استاد سید مهدی سیف درباره موضوع حروف ناخوانا و الف بعد از واو آخر کلمه توضیح می دهند همچنین به مرور دروس گذشته می پردازند.وارد الفالف در…